README.md
1# HiSpark WiFi-IoT 开发套件样例开发--线程(Thread)
2
3
4
5[HiSpark WiFi-IoT开发套件]首发于HDC 2020,是首批支持OpenHarmony 2.0的开发套件,亦是官方推荐套件,由润和软件HiHope量身打造,已在OpenHarmony社区和广大OpenHarmony开发者中得到广泛应用。
6
7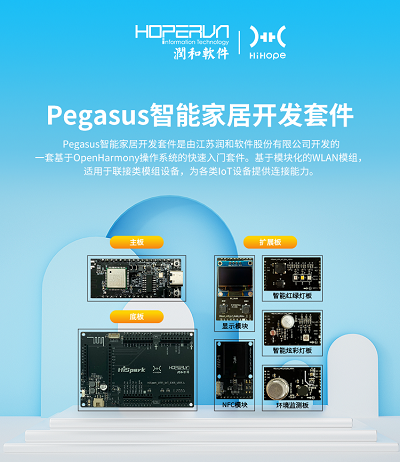
8
9## 一、Thread API
10
11| API名称 | 说明 |
12| --------------------- | ------------------------------------------------------ |
13| osThreadNew | 创建一个线程并将其加入活跃线程组中 |
14| osThreadGetName | 返回指定线程的名字 |
15| osThreadGetId | 返回当前运行线程的线程ID |
16| osThreadGetState | 返回当前线程的状态 |
17| osThreadSetPriority | 设置指定线程的优先级 |
18| osThreadGetPriority | 获取当前线程的优先级 |
19| osThreadYield | 将运行控制转交给下一个处于READY状态的线程 |
20| osThreadSuspend | 挂起指定线程的运行 |
21| osThreadResume | 恢复指定线程的运行 |
22| osThreadDetach | 分离指定的线程(当线程终止运行时,线程存储可以被回收) |
23| osThreadJoin | 等待指定线程终止运行 |
24| osThreadExit | 终止当前线程的运行 |
25| osThreadTerminate | 终止指定线程的运行 |
26| osThreadGetStackSize | 获取指定线程的栈空间大小 |
27| osThreadGetStackSpace | 获取指定线程的未使用的栈空间大小 |
28| osThreadGetCount | 获取活跃线程数 |
29| osThreadEnumerate | 获取线程组中的活跃线程数 |
30
31### osThreadNew()
32
33```c
34osThreadId_t osThreadNew(osThreadFunc_t func, void *argument,const osThreadAttr_t *attr )
35```
36
37> **注意** :不能在中断服务调用该函数
38
39**参数:**
40
41| 名字 | 描述 |
42| :------- | :------------------------------- |
43| func | 线程函数. |
44| argument | 作为启动参数传递给线程函数的指针 |
45| attr | 线程属性 |
46
47### osThreadTerminate()
48
49```c
50osStatus_t osThreadTerminate (osThreadId_t thread_id)
51```
52
53| 名字 | 描述 |
54| --------- | ---------------------------------------------------- |
55| thread_id | 指定线程id,该id是由osThreadNew或者osThreadGetId获得 |
56
57## 二、代码分析
58
59创建线程,创建成功则打印线程名字和线程ID
60
61```
62osThreadId_t newThread(char *name, osThreadFunc_t func, void *arg) {
63 osThreadAttr_t attr = {
64 name, 0, NULL, 0, NULL, 1024*2, osPriorityNormal, 0, 0
65 };
66 osThreadId_t tid = osThreadNew(func, arg, &attr);
67 if (tid == NULL) {
68 printf("osThreadNew(%s) failed.\r\n", name);
69 } else {
70 printf("osThreadNew(%s) success, thread id: %d.\r\n", name, tid);
71 }
72 return tid;
73}
74```
75
76该函数首先会打印自己的参数,然后对全局变量count进行循环+1操作,之后会打印count的值
77
78```
79void threadTest(void *arg) {
80 static int count = 0;
81 printf("%s\r\n",(char *)arg);
82 osThreadId_t tid = osThreadGetId();
83 printf("threadTest osThreadGetId, thread id:%p\r\n", tid);
84 while (1) {
85 count++;
86 printf("threadTest, count: %d.\r\n", count);
87 osDelay(20);
88 }
89}
90```
91
92主程序rtosv2_thread_main创建线程并运行,并使用上述API进行相关操作,最后终止所创建的线程。
93
94```
95void rtosv2_thread_main(void *arg) {
96 (void)arg;
97 osThreadId_t tid=newThread("test_thread", threadTest, "This is a test thread.");
98
99 const char *t_name = osThreadGetName(tid);
100 printf("[Thread Test]osThreadGetName, thread name: %s.\r\n", t_name);
101
102 osThreadState_t state = osThreadGetState(tid);
103 printf("[Thread Test]osThreadGetState, state :%d.\r\n", state);
104
105 osStatus_t status = osThreadSetPriority(tid, osPriorityNormal4);
106 printf("[Thread Test]osThreadSetPriority, status: %d.\r\n", status);
107
108 osPriority_t pri = osThreadGetPriority (tid);
109 printf("[Thread Test]osThreadGetPriority, priority: %d.\r\n", pri);
110
111 status = osThreadSuspend(tid);
112 printf("[Thread Test]osThreadSuspend, status: %d.\r\n", status);
113
114 status = osThreadResume(tid);
115 printf("[Thread Test]osThreadResume, status: %d.\r\n", status);
116
117 uint32_t stacksize = osThreadGetStackSize(tid);
118 printf("[Thread Test]osThreadGetStackSize, stacksize: %d.\r\n", stacksize);
119
120 uint32_t stackspace = osThreadGetStackSpace(tid);
121 printf("[Thread Test]osThreadGetStackSpace, stackspace: %d.\r\n", stackspace);
122
123 uint32_t t_count = osThreadGetCount();
124 printf("[Thread Test]osThreadGetCount, count: %d.\r\n", t_count);
125
126 osDelay(100);
127 status = osThreadTerminate(tid);
128 printf("[Thread Test]osThreadTerminate, status: %d.\r\n", status);
129}
130```
131
132## 三、如何编译
133
1341. 将此目录下的 `thread.c` 和 `BUILD.gn` 复制到openharmony源码的`applications\sample\wifi-iot\app\iothardware`目录下,
1352. 修改openharmony源码的`applications\sample\wifi-iot\app\BUILD.gn`文件,将其中的 `features` 改为:
136
137```
138 features = [
139 "iothardware:thread_demo",
140 ]
141```
142
1433. 在openharmony源码顶层目录执行:`python build.py wifiiot`
144
145## 四、运行结果
146
147```
148[Thread Test] osThreadNew(test_thread) success.
149[Thread Test] osThreadGetName, thread name: test_thread.
150[Thread Test] osThreadGetState, state :1.
151[Thread Test] This is a test thread. <-testThread log
152[Thread Test] threadTest osThreadGetId, thread id:0xe8544
153[Thread Test] threadTest, count: 1. <-testThread log
154[Thread Test] osThreadSetPriority, status: 0.
155[Thread Test] osThreadGetPriority, priority: 28.
156[Thread Test] osThreadSuspend, status: 0.
157[Thread Test] osThreadResume, status: 0.
158[Thread Test] osThreadGetStackSize, stacksize: 2048.
159[Thread Test] osThreadGetStackSpace, stackspace: 1144.
160[Thread Test] osThreadGetCount, count: 12.
161[Thread Test] threadTest, count: 2. <-testThread log
162[Thread Test] threadTest, count: 3. <-testThread log
163[Thread Test] threadTest, count: 4. <-testThread log
164[Thread Test] threadTest, count: 5. <-testThread log
165[Thread Test] threadTest, count: 6. <-testThread log
166[Thread Test] osThreadTerminate, status: 0.
167```
168
169### 【套件支持】
170
171##### 1. 套件介绍 http://www.hihope.org/pro/pro1.aspx?mtt=8
172
173##### 2. 套件购买 https://item.taobao.com/item.htm?id=622343426064&scene=taobao_shop
174
175##### 3. 技术资料
176
177- Gitee码云网站(OpenHarmony Sample Code等) **https://gitee.com/hihopeorg**
178
179- HiHope官网-资源中心(SDK包、技术文档下载)[**www.hihope.org**](http://www.hihope.org/)
180
181##### 4. 互动交流
182
183- 润和HiHope技术交流-微信群(加群管理员微信13605188699,发送文字#申请加入润和官方OpenHarmony群#,予以邀请入群)
184- HiHope开发者社区-论坛 **https://bbs.elecfans.com/group_1429**
185- 润和HiHope售后服务群(QQ:980599547)
186- 售后服务电话(025-52668590)
187
188