README.OpenSource
README.md
1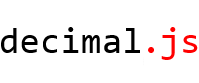
2
3An arbitrary-precision Decimal type for JavaScript.
4
5[](https://www.npmjs.com/package/decimal.js)
6[](https://www.npmjs.com/package/decimal.js)
7[](https://travis-ci.org/MikeMcl/decimal.js)
8[](https://cdnjs.com/libraries/decimal.js)
9
10<br>
11
12## Features
13
14 - Integers and floats
15 - Simple but full-featured API
16 - Replicates many of the methods of JavaScript's `Number.prototype` and `Math` objects
17 - Also handles hexadecimal, binary and octal values
18 - Faster, smaller, and perhaps easier to use than JavaScript versions of Java's BigDecimal
19 - No dependencies
20 - Wide platform compatibility: uses JavaScript 1.5 (ECMAScript 3) features only
21 - Comprehensive [documentation](https://mikemcl.github.io/decimal.js/) and test set
22 - Used under the hood by [math.js](https://github.com/josdejong/mathjs)
23 - Includes a TypeScript declaration file: *decimal.d.ts*
24
25
26
27The library is similar to [bignumber.js](https://github.com/MikeMcl/bignumber.js/), but here
28precision is specified in terms of significant digits rather than decimal places, and all
29calculations are rounded to the precision (similar to Python's decimal module) rather than just
30those involving division.
31
32This library also adds the trigonometric functions, among others, and supports non-integer powers,
33which makes it a significantly larger library than *bignumber.js* and the even smaller
34[big.js](https://github.com/MikeMcl/big.js/).
35
36For a lighter version of this library without the trigonometric functions see
37[decimal.js-light](https://github.com/MikeMcl/decimal.js-light/).
38
39## Load
40
41The library is the single JavaScript file *decimal.js* or ES module *decimal.mjs*.
42
43Browser:
44
45```html
46<script src='path/to/decimal.js'></script>
47
48<script type="module">
49 import Decimal from './path/to/decimal.mjs';
50 ...
51</script>
52```
53
54[Node.js](https://nodejs.org):
55
56```bash
57npm install decimal.js
58```
59```js
60const Decimal = require('decimal.js');
61
62import Decimal from 'decimal.js';
63
64import {Decimal} from 'decimal.js';
65```
66
67## Use
68
69*In all examples below, semicolons and `toString` calls are not shown.
70If a commented-out value is in quotes it means `toString` has been called on the preceding expression.*
71
72The library exports a single constructor function, `Decimal`, which expects a single argument that is a number, string or Decimal instance.
73
74```js
75x = new Decimal(123.4567)
76y = new Decimal('123456.7e-3')
77z = new Decimal(x)
78x.equals(y) && y.equals(z) && x.equals(z) // true
79```
80
81If using values with more than a few digits, it is recommended to pass strings rather than numbers to avoid a potential loss of precision.
82
83```js
84// Precision loss from using numeric literals with more than 15 significant digits.
85new Decimal(1.0000000000000001) // '1'
86new Decimal(88259496234518.57) // '88259496234518.56'
87new Decimal(99999999999999999999) // '100000000000000000000'
88
89// Precision loss from using numeric literals outside the range of Number values.
90new Decimal(2e+308) // 'Infinity'
91new Decimal(1e-324) // '0'
92
93// Precision loss from the unexpected result of arithmetic with Number values.
94new Decimal(0.7 + 0.1) // '0.7999999999999999'
95```
96
97As with JavaScript numbers, strings can contain underscores as separators to improve readability.
98
99```js
100x = new Decimal('2_147_483_647')
101```
102
103String values in binary, hexadecimal or octal notation are also accepted if the appropriate prefix is included.
104
105```js
106x = new Decimal('0xff.f') // '255.9375'
107y = new Decimal('0b10101100') // '172'
108z = x.plus(y) // '427.9375'
109
110z.toBinary() // '0b110101011.1111'
111z.toBinary(13) // '0b1.101010111111p+8'
112
113// Using binary exponential notation to create a Decimal with the value of `Number.MAX_VALUE`.
114x = new Decimal('0b1.1111111111111111111111111111111111111111111111111111p+1023')
115// '1.7976931348623157081e+308'
116```
117
118Decimal instances are immutable in the sense that they are not changed by their methods.
119
120```js
1210.3 - 0.1 // 0.19999999999999998
122x = new Decimal(0.3)
123x.minus(0.1) // '0.2'
124x // '0.3'
125```
126
127The methods that return a Decimal can be chained.
128
129```js
130x.dividedBy(y).plus(z).times(9).floor()
131x.times('1.23456780123456789e+9').plus(9876.5432321).dividedBy('4444562598.111772').ceil()
132```
133
134Many method names have a shorter alias.
135
136```js
137x.squareRoot().dividedBy(y).toPower(3).equals(x.sqrt().div(y).pow(3)) // true
138x.comparedTo(y.modulo(z).negated() === x.cmp(y.mod(z).neg()) // true
139```
140
141Most of the methods of JavaScript's `Number.prototype` and `Math` objects are replicated.
142
143```js
144x = new Decimal(255.5)
145x.toExponential(5) // '2.55500e+2'
146x.toFixed(5) // '255.50000'
147x.toPrecision(5) // '255.50'
148
149Decimal.sqrt('6.98372465832e+9823') // '8.3568682281821340204e+4911'
150Decimal.pow(2, 0.0979843) // '1.0702770511687781839'
151
152// Using `toFixed()` to avoid exponential notation:
153x = new Decimal('0.0000001')
154x.toString() // '1e-7'
155x.toFixed() // '0.0000001'
156```
157
158And there are `isNaN` and `isFinite` methods, as `NaN` and `Infinity` are valid `Decimal` values.
159
160```js
161x = new Decimal(NaN) // 'NaN'
162y = new Decimal(Infinity) // 'Infinity'
163x.isNaN() && !y.isNaN() && !x.isFinite() && !y.isFinite() // true
164```
165
166There is also a `toFraction` method with an optional *maximum denominator* argument.
167
168```js
169z = new Decimal(355)
170pi = z.dividedBy(113) // '3.1415929204'
171pi.toFraction() // [ '7853982301', '2500000000' ]
172pi.toFraction(1000) // [ '355', '113' ]
173```
174
175All calculations are rounded according to the number of significant digits and rounding mode specified
176by the `precision` and `rounding` properties of the Decimal constructor.
177
178For advanced usage, multiple Decimal constructors can be created, each with their own independent
179configuration which applies to all Decimal numbers created from it.
180
181```js
182// Set the precision and rounding of the default Decimal constructor
183Decimal.set({ precision: 5, rounding: 4 })
184
185// Create another Decimal constructor, optionally passing in a configuration object
186Dec = Decimal.clone({ precision: 9, rounding: 1 })
187
188x = new Decimal(5)
189y = new Dec(5)
190
191x.div(3) // '1.6667'
192y.div(3) // '1.66666666'
193```
194
195The value of a Decimal is stored in a floating point format in terms of its digits, exponent and sign, but these properties should be considered read-only.
196
197```js
198x = new Decimal(-12345.67);
199x.d // [ 12345, 6700000 ] digits (base 10000000)
200x.e // 4 exponent (base 10)
201x.s // -1 sign
202```
203
204For further information see the [API](http://mikemcl.github.io/decimal.js/) reference in the *doc* directory.
205
206## Test
207
208To run the tests using Node.js from the root directory:
209
210```bash
211npm test
212```
213
214Each separate test module can also be executed individually, for example:
215
216```bash
217node test/modules/toFraction
218```
219
220To run the tests in a browser, open *test/test.html*.
221
222## Minify
223
224Two minification examples:
225
226Using [uglify-js](https://github.com/mishoo/UglifyJS) to minify the *decimal.js* file:
227
228```bash
229npm install uglify-js -g
230uglifyjs decimal.js --source-map url=decimal.min.js.map -c -m -o decimal.min.js
231```
232
233Using [terser](https://github.com/terser/terser) to minify the ES module version, *decimal.mjs*:
234
235```bash
236npm install terser -g
237terser decimal.mjs --source-map url=decimal.min.mjs.map -c -m --toplevel -o decimal.min.mjs
238```
239
240```js
241import Decimal from './decimal.min.mjs';
242```
243
244## Licence
245
246[The MIT Licence (Expat).](LICENCE.md)
247