README.md
1# HiSpark WiFi-IoT 套件样例开发--wificonnect(STA模式)
2
3
4
5[HiSpark WiFi-IoT开发套件](https://item.taobao.com/item.htm?id=622343426064&scene=taobao_shop) 首发于HDC 2020,是首批支持OpenHarmony 2.0的开发套件,亦是官方推荐套件,由润和软件HiHope量身打造,已在OpenHarmony社区和广大OpenHarmony开发者中得到广泛应用。
6
7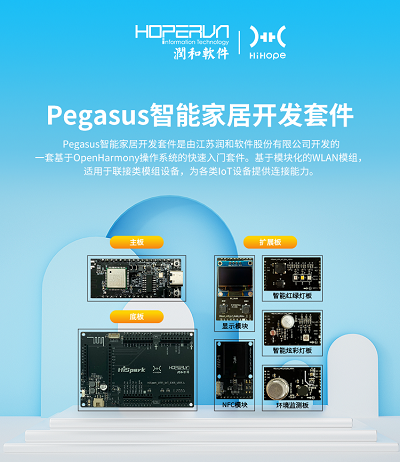
8
9## 一、Wifi STA API
10
11使用原始WiFI API接口进行编程,STA模式需要使用原始STA接口以及一些DHCP客户端接口。
12
13#### WiFi STA模式相关的API接口文件路径
14
15**foundation/communication/interfaces/kits/wifi_lite/wifiservice/wifi_device.h**
16所使用的API接口有:
17
18
19| API | 接口说明 |
20| ------------------------------------------------------------ | --------------------------------------- |
21| WifiErrorCode EnableWifi(void); | 开启STA |
22| WifiErrorCode DisableWifi(void); | 关闭STA |
23| int IsWifiActive(void); | 查询STA是否已开启 |
24| WifiErrorCode Scan(void); | 触发扫描 |
25| WifiErrorCode GetScanInfoList(WifiScanInfo* result, unsigned int* size); | 获取扫描结果 |
26| WifiErrorCode AddDeviceConfig(const WifiDeviceConfig* config, int* result); | 添加热点配置,成功会通过result传出netld |
27| WifiErrorCode GetDeviceConfigs(WifiDeviceConfig* result, unsigned int* size); | 获取本机所有热点配置 |
28| WifiErrorCode RemoveDevice(int networkId); | 删除热点配置 |
29| WifiErrorCode ConnectTo(int networkId); | 连接到热点 |
30| WifiErrorCode Disconnect(void); | 断开热点连接 |
31| WifiErrorCode GetLinkedInfo(WifiLinkedInfo* result); | 获取当前连接热点信息 |
32| WifiErrorCode RegisterWifiEvent(WifiEvent* event); | 注册事件监听 |
33| WifiErrorCode UnRegisterWifiEvent(const WifiEvent* event); | 解除事件监听 |
34| WifiErrorCode GetDeviceMacAddress(unsigned char* result); | 获取Mac地址 |
35| WifiErrorCode AdvanceScan(WifiScanParams *params); | 高级搜索 |
36
37#### Hi3861 SDK的DHCP客户端接口:
38
39| API | 描述 |
40| ------------------- | ------------------ |
41| netifapi_netif_find | 按名称查找网络接口 |
42| netifapi_dhcp_start | 启动DHCP客户端 |
43| netifapi_dhcp_stop | 停止DHCP客户端 |
44
45## 二、代码分析
46
47```c
48//wifi connect task
49static void WifiConnectTask(void *arg)
50{
51 (void)arg;
52 WifiErrorCode errCode;
53 WifiEvent eventListener = {
54 .OnWifiConnectionChanged = OnWifiConnectionChanged,
55 .OnWifiScanStateChanged = OnWifiScanStateChanged
56 };
57 WifiDeviceConfig apConfig = {};
58 int netId = -1;
59
60 osDelay(10);
61 errCode = RegisterWifiEvent(&eventListener);
62 printf("RegisterWifiEvent: %d\r\n", errCode);
63
64 // 配置要连接的热点的账号密码
65 strcpy(apConfig.ssid, "ABCD");
66 strcpy(apConfig.preSharedKey, "12345678");
67 apConfig.securityType = WIFI_SEC_TYPE_PSK;
68
69 while (1) {
70 //打开wifi
71 errCode = EnableWifi();
72 printf("EnableWifi: %d\r\n", errCode);
73 osDelay(11);
74 //添加热点配置
75 errCode = AddDeviceConfig(&apConfig, &netId);
76 printf("AddDeviceConfig: %d\r\n", errCode);
77
78 g_connected = 0;
79 //连接热点
80 errCode = ConnectTo(netId);
81 printf("ConnectTo(%d): %d\r\n", netId, errCode);
82 //等待wifi连接
83 while (!g_connected) {
84 osDelay(10);
85 }
86 printf("g_connected: %d\r\n", g_connected);
87 osDelay(50);
88
89
90 // 联网业务开始
91 struct netif* iface = netifapi_netif_find("wlan0");
92 if (iface) {
93 err_t ret = netifapi_dhcp_start(iface);
94 printf("netifapi_dhcp_start: %d\r\n", ret);
95
96 osDelay(200); // wait DHCP server give me IP
97 ret = netifapi_netif_common(iface, dhcp_clients_info_show, NULL);
98 printf("netifapi_netif_common: %d\r\n", ret);
99 }
100
101 // 模拟一段时间的联网业务
102 int timeout = 60;
103 while (timeout--) {
104 osDelay(100);
105 printf("after %d seconds, I'll disconnect WiFi!\n", timeout);
106 }
107
108 // 联网业务结束
109 err_t ret = netifapi_dhcp_stop(iface);
110 printf("netifapi_dhcp_stop: %d\r\n", ret);
111
112 Disconnect(); // disconnect with your AP
113
114 RemoveDevice(netId); // remove AP config
115
116 errCode = DisableWifi(); //close wifi
117 printf("DisableWifi: %d\r\n", errCode);
118 osDelay(200);
119 }
120}
121
122```
123
124
125
126## 三、如何编译
127
1281. 将此目录下的 `wifi_connect_demo.c` 和 `BUILD.gn` 复制到openharmony源码的`applications\sample\wifi-iot\app\iothardware`目录下,
1292. 修改openharmony源码的`applications\sample\wifi-iot\app\BUILD.gn`文件,将其中的 `features` 改为:
130
131```
132 features = [
133 "iothardware:wifi_demo",
134 ]
135```
136
1373. 在openharmony源码顶层目录执行:`python build.py wifiiot`
138
139## 四、运行结果
140
141```
142
143ready to OS start
144sdk ver:Hi3861V100R001C00SPC025 2020-09-03 18:10:00
145FileSystem mount ok.
146wifi init success!
147
14800 00:00:00 0 132 D 0/HIVIEW: hilog init success.
14900 00:00:00 0 132 D 0/HIVIEW: log limit init success.
15000 00:00:00 0 132 I 1/SAMGR: Bootstrap core services(count:3).
15100 00:00:00 0 132 I 1/SAMGR: Init service:0x4af3e8 TaskPool:0xe4f38
15200 00:00:00 0 132 I 1/SAMGR: Init service:0x4af3f4 TaskPool:0xe4f58
15300 00:00:00 0 132 I 1/SAMGR: Init service:0x4af51c TaskPool:0xe4f78
15400 00:00:00 0 164 I 1/SAMGR: Init service 0x4af3f4 <time: 0ms> success!
15500 00:00:00 0 64 I 1/SAMGR: Init service 0x4af3e8 <time: 0ms> success!
15600 00:00:00 0 8 D 0/HIVIEW: hiview init success.
15700 00:00:00 0 8 I 1/SAMGR: Init service 0x4af51c <time: 0ms> success!
15800 00:00:00 0 8 I 1/SAMGR: Initialized all core system services!
15900 00:00:00 0 64 I 1/SAMGR: Bootstrap system and application services(count:0).
16000 00:00:00 0 64 I 1/SAMGR: Initialized all system and application services!
16100 00:00:00 0 64 I 1/SAMGR: Bootstrap dynamic registered services(count:0).
162RegisterWifiEvent: 0
163EnableWifi: 0
164AddDeviceConfig: 0
165ConnectTo(0): 0
166+NOTICE:SCANFINISH
167+NOTICE:CONNECTED
168OnWifiConnectionChanged 58, state = 1, info =
169bssid: 08:1F:71:24:B8:29, rssi: 0, connState: 0, reason: 0, ssid: ABCD
170g_connected: 1
171netifapi_dhcp_start: 0
172server :
173 server_id : 192.168.0.1
174 mask : 255.255.255.0, 1
175 gw : 192.168.0.1
176 T0 : 7200
177 T1 : 3600
178 T2 : 6300
179clients <1> :
180 mac_idx mac addr state lease tries rto
181 0 b4c9b96199d6 192.168.0.107 10 0 1 2
182netifapi_netif_common: 0
183after 59 seconds, I'll disconnect WiFi!
184after 58 seconds, I'll disconnect WiFi!
185after 57 seconds, I'll disconnect WiFi!
186...
187...
188...
189after 3 seconds, I'll disconnect WiFi!
190after 2 seconds, I'll disconnect WiFi!
191after 1 seconds, I'll disconnect WiFi!
192after 0 seconds, I'll disconnect WiFi!
193netifapi_dhcp_stop: 0
194+NOTICE:DISCONNECTED
195OnWifiConnectionChanged 58, state = 0, info =
196bssid: 08:1F:71:24:B8:29, rssi: 0, connState: 0, reason: 3, ssid:
197DisableWifi: 0
198
199```
200
201
202
203
204
205### 【套件支持】
206
207##### 1. 套件介绍 http://www.hihope.org/pro/pro1.aspx?mtt=8
208
209##### 2. 套件购买 https://item.taobao.com/item.htm?id=622343426064&scene=taobao_shop
210
211##### 3. 技术资料
212
213- Gitee码云网站(OpenHarmony Sample Code等) **https://gitee.com/hihopeorg**
214
215- HiHope官网-资源中心(SDK包、技术文档下载)**http://www.hihope.org/**
216
217##### 4. 互动交流
218
219- 润和HiHope技术交流-微信群(加群管理员微信13605188699,发送文字#申请加入润和官方群#,予以邀请入群)
220- HiHope开发者社区-论坛 **https://bbs.elecfans.com/group_1429**
221- 润和HiHope售后服务群(QQ:980599547)
222- 售后服务电话(025-52668590)
223