1 //! [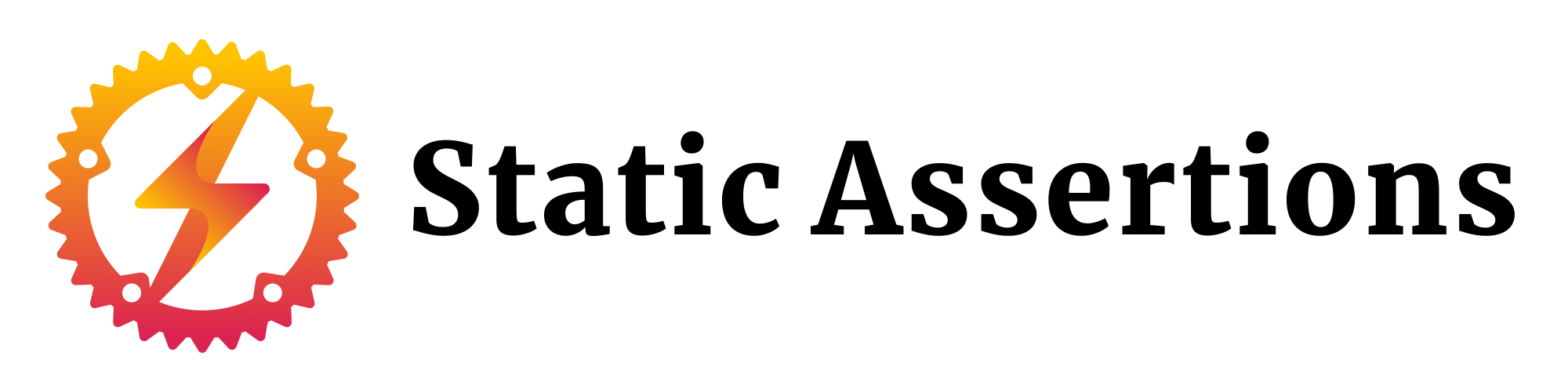](https://github.com/nvzqz/static-assertions-rs) 2 //! 3 //! <div align="center"> 4 //! <a href="https://crates.io/crates/static_assertions"> 5 //! <img src="https://img.shields.io/crates/d/static_assertions.svg" alt="Downloads"> 6 //! </a> 7 //! <a href="https://travis-ci.org/nvzqz/static-assertions-rs"> 8 //! <img src="https://travis-ci.org/nvzqz/static-assertions-rs.svg?branch=master" alt="Build Status"> 9 //! </a> 10 //! <img src="https://img.shields.io/badge/rustc-^1.37.0-blue.svg" alt="rustc ^1.37.0"> 11 //! <br><br> 12 //! </div> 13 //! 14 //! Assertions to ensure correct assumptions about constants, types, and more. 15 //! 16 //! _All_ checks provided by this crate are performed at [compile-time]. This 17 //! allows for finding errors quickly and early when it comes to ensuring 18 //! certain features or aspects of a codebase. These macros are especially 19 //! important when exposing a public API that requires types to be the same size 20 //! or implement certain traits. 21 //! 22 //! # Usage 23 //! 24 //! This crate is available [on crates.io][crate] and can be used by adding the 25 //! following to your project's [`Cargo.toml`]: 26 //! 27 //! ```toml 28 //! [dependencies] 29 //! static_assertions = "1.1.0" 30 //! ``` 31 //! 32 //! and this to your crate root (`main.rs` or `lib.rs`): 33 //! 34 //! ``` 35 //! #[macro_use] 36 //! extern crate static_assertions; 37 //! # fn main() {} 38 //! ``` 39 //! 40 //! When using [Rust 2018 edition][2018], the following shorthand can help if 41 //! having `#[macro_use]` is undesirable. 42 //! 43 //! ```edition2018 44 //! extern crate static_assertions as sa; 45 //! 46 //! sa::const_assert!(true); 47 //! ``` 48 //! 49 //! # Examples 50 //! 51 //! Very thorough examples are provided in the docs for 52 //! [each individual macro](#macros). Failure case examples are also documented. 53 //! 54 //! # Changes 55 //! 56 //! See [`CHANGELOG.md`](https://github.com/nvzqz/static-assertions-rs/blob/master/CHANGELOG.md) 57 //! for an exhaustive list of what has changed from one version to another. 58 //! 59 //! # Donate 60 //! 61 //! This project is made freely available (as in free beer), but unfortunately 62 //! not all beer is free! So, if you would like to buy me a beer (or coffee or 63 //! *more*), then consider supporting my work that's benefited your project 64 //! and thousands of others. 65 //! 66 //! <a href="https://www.patreon.com/nvzqz"> 67 //! <img src="https://c5.patreon.com/external/logo/become_a_patron_button.png" alt="Become a Patron!" height="35"> 68 //! </a> 69 //! <a href="https://www.paypal.me/nvzqz"> 70 //! <img src="https://buymecoffee.intm.org/img/button-paypal-white.png" alt="Buy me a coffee" height="35"> 71 //! </a> 72 //! 73 //! [Rust 1.37]: https://blog.rust-lang.org/2019/08/15/Rust-1.37.0.html 74 //! [2018]: https://blog.rust-lang.org/2018/12/06/Rust-1.31-and-rust-2018.html#rust-2018 75 //! [crate]: https://crates.io/crates/static_assertions 76 //! [compile-time]: https://en.wikipedia.org/wiki/Compile_time 77 //! [`Cargo.toml`]: https://doc.rust-lang.org/cargo/reference/manifest.html 78 79 #![doc(html_root_url = "https://docs.rs/static_assertions/1.1.0")] 80 #![doc(html_logo_url = "https://raw.githubusercontent.com/nvzqz/static-assertions-rs/assets/Icon.png")] 81 82 #![no_std] 83 84 #![deny(unused_macros)] 85 86 #[doc(hidden)] 87 pub extern crate core as _core; 88 89 mod assert_cfg; 90 mod assert_eq_align; 91 mod assert_eq_size; 92 mod assert_fields; 93 mod assert_impl; 94 mod assert_obj_safe; 95 mod assert_trait; 96 mod assert_type; 97 mod const_assert; 98