1# Mojo 2 3[TOC] 4 5## Getting Started With Mojo 6 7To get started using Mojo in applications which already support it (such as 8Chrome), the fastest path forward will be to look at the bindings documentation 9for your language of choice ([**C++**](#C_Bindings), 10[**JavaScript**](#JavaScript-Bindings), or [**Java**](#Java-Bindings)) as well 11as the documentation for the 12[**Mojom IDL and bindings generator**](/mojo/public/tools/bindings/README.md). 13 14If you're looking for information on creating and/or connecting to services, see 15the top-level [Services documentation](/services/README.md). 16 17For specific details regarding the conversion of old things to new things, check 18out [Converting Legacy Chrome IPC To Mojo](/ipc/README.md). 19 20## System Overview 21 22Mojo is a collection of runtime libraries providing a platform-agnostic 23abstraction of common IPC primitives, a message IDL format, and a bindings 24library with code generation for multiple target languages to facilitate 25convenient message passing across arbitrary inter- and intra-process boundaries. 26 27The documentation here is segmented according to the different libraries 28comprising Mojo. The basic hierarchy of features is as follows: 29 30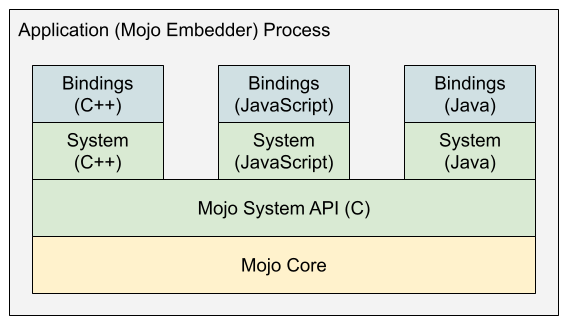 31 32## Mojo Core 33In order to use any of the more interesting high-level support libraries like 34the System APIs or Bindings APIs, a process must first initialize Mojo Core. 35This is a one-time initialization which remains active for the remainder of the 36process's lifetime. There are two ways to initialize Mojo Core: via the Embedder 37API, or through a dynamically linked library. 38 39### Embedding 40Many processes to be interconnected via Mojo are **embedders**, meaning that 41they statically link against the `//mojo/core/embedder` target and initialize 42Mojo support within each process by calling `mojo::core::Init()`. See 43[**Mojo Core Embedder API**](/mojo/core/embedder/README.md) for more details. 44 45This is a reasonable option when you can guarantee that all interconnected 46process binaries are linking against precisely the same revision of Mojo Core. 47To support other scenarios, use dynamic linking. 48 49## Dynamic Linking 50On some platforms, it's also possible for applications to rely on a 51dynamically-linked Mojo Core library (`libmojo_core.so` or `mojo_core.dll`) 52instead of statically linking against Mojo Core. 53 54In order to take advantage of this mechanism, the corresponding library must be 55present in either: 56 57 - The working directory of the application 58 - A directory named by the `MOJO_CORE_LIBRARY_PATH` environment variable 59 - A directory named explicitly by the application at runtime 60 61Instead of calling `mojo::core::Init()` as embedders do, an application using 62dynamic Mojo Core instead calls `MojoInitialize()` from the C System API. This 63call will attempt to locate (see above) and load a Mojo Core library to support 64subsequent Mojo API usage within the process. 65 66Note that the Mojo Core shared library presents a stable, forward-compatible C 67ABI which can support all current and future versions of the higher-level, 68public (and not binary-stable) System and Bindings APIs. 69 70## C System API 71Once Mojo is initialized within a process, the public 72[**C System API**](/mojo/public/c/system/README.md) is usable on any thread for 73the remainder of the process's lifetime. This is a lightweight API with a 74relatively small, stable, forward-compatible ABI, comprising the total public 75API surface of the Mojo Core library. 76 77This API is rarely used directly, but it is the foundation upon which all 78higher-level Mojo APIs are built. It exposes the fundamental capabilities to 79create and interact Mojo primitives like **message pipes**, **data pipes**, and 80**shared buffers**, as well as APIs to help bootstrap connections among 81processes. 82 83## Platform Support API 84Mojo provides a small collection of abstractions around platform-specific IPC 85primitives to facilitate bootstrapping Mojo IPC between two processes. See the 86[Platform API](/mojo/public/cpp/platform/README.md) documentation for details. 87 88## High-Level System APIs 89There is a relatively small, higher-level system API for each supported 90language, built upon the low-level C API. Like the C API, direct usage of these 91system APIs is rare compared to the bindings APIs, but it is sometimes desirable 92or necessary. 93 94### C++ 95The [**C++ System API**](/mojo/public/cpp/system/README.md) provides a layer of 96C++ helper classes and functions to make safe System API usage easier: 97strongly-typed handle scopers, synchronous waiting operations, system handle 98wrapping and unwrapping helpers, common handle operations, and utilities for 99more easily watching handle state changes. 100 101### JavaScript 102The [**JavaScript System API**](/third_party/blink/renderer/core/mojo/README.md) 103exposes the Mojo primitives to JavaScript, covering all basic functionality of the 104low-level C API. 105 106### Java 107The [**Java System API**](/mojo/public/java/system/README.md) provides helper 108classes for working with Mojo primitives, covering all basic functionality of 109the low-level C API. 110 111## High-Level Bindings APIs 112Typically developers do not use raw message pipe I/O directly, but instead 113define some set of interfaces which are used to generate code that resembles 114an idiomatic method-calling interface in the target language of choice. This is 115the bindings layer. 116 117### Mojom IDL and Bindings Generator 118Interfaces are defined using the 119[**Mojom IDL**](/mojo/public/tools/bindings/README.md), which can be fed to the 120[**bindings generator**](/mojo/public/tools/bindings/README.md) to generate code 121in various supported languages. Generated code manages serialization and 122deserialization of messages between interface clients and implementations, 123simplifying the code -- and ultimately hiding the message pipe -- on either side 124of an interface connection. 125 126### C++ Bindings 127By far the most commonly used API defined by Mojo, the 128[**C++ Bindings API**](/mojo/public/cpp/bindings/README.md) exposes a robust set 129of features for interacting with message pipes via generated C++ bindings code, 130including support for sets of related bindings endpoints, associated interfaces, 131nested sync IPC, versioning, bad-message reporting, arbitrary message filter 132injection, and convenient test facilities. 133 134### JavaScript Bindings 135The [**JavaScript Bindings API**](/mojo/public/js/README.md) provides helper 136classes for working with JavaScript code emitted by the bindings generator. 137 138### Java Bindings 139The [**Java Bindings API**](/mojo/public/java/bindings/README.md) provides 140helper classes for working with Java code emitted by the bindings generator. 141 142## FAQ 143 144### Why not protobuf? Why a new thing? 145There are number of potentially decent answers to this question, but the 146deal-breaker is that a useful IPC mechanism must support transfer of native 147object handles (*e.g.* file descriptors) across process boundaries. Other 148non-new IPC things that do support this capability (*e.g.* D-Bus) have their own 149substantial deficiencies. 150 151### Are message pipes expensive? 152No. As an implementation detail, creating a message pipe is essentially 153generating two random numbers and stuffing them into a hash table, along with a 154few tiny heap allocations. 155 156### So really, can I create like, thousands of them? 157Yes! Nobody will mind. Create millions if you like. (OK but maybe don't.) 158 159### What are the performance characteristics of Mojo? 160Compared to the old IPC in Chrome, making a Mojo call is about 1/3 faster and uses 1611/3 fewer context switches. The full data is [available here](https://docs.google.com/document/d/1n7qYjQ5iy8xAkQVMYGqjIy_AXu2_JJtMoAcOOupO_jQ/edit). 162 163### Can I use in-process message pipes? 164Yes, and message pipe usage is identical regardless of whether the pipe actually 165crosses a process boundary -- in fact this detail is intentionally obscured. 166 167Message pipes which don't cross a process boundary are efficient: sent messages 168are never copied, and a write on one end will synchronously modify the message 169queue on the other end. When working with generated C++ bindings, for example, 170the net result is that an `InterfacePtr` on one thread sending a message to a 171`Binding` on another thread (or even the same thread) is effectively a 172`PostTask` to the `Binding`'s `TaskRunner` with the added -- but often small -- 173costs of serialization, deserialization, validation, and some internal routing 174logic. 175 176### What about ____? 177 178Please post questions to 179[`chromium-mojo@chromium.org`](https://groups.google.com/a/chromium.org/forum/#!forum/chromium-mojo)! 180The list is quite responsive. 181 182