1 /*! 2 Defines the drawing elements, the high-level drawing unit in Plotters drawing system 3 4 ## Introduction 5 An element is the drawing unit for Plotter's high-level drawing API. 6 Different from low-level drawing API, an element is a logic unit of component in the image. 7 There are few built-in elements, including `Circle`, `Pixel`, `Rectangle`, `Path`, `Text`, etc. 8 9 All element can be drawn onto the drawing area using API `DrawingArea::draw(...)`. 10 Plotters use "iterator of elements" as the abstraction of any type of plot. 11 12 ## Implementing your own element 13 You can also define your own element, `CandleStick` is a good sample of implementing complex 14 element. There are two trait required for an element: 15 16 - `PointCollection` - the struct should be able to return an iterator of key-points under guest coordinate 17 - `Drawable` - the struct is a pending drawing operation on a drawing backend with pixel-based coordinate 18 19 An example of element that draws a red "X" in a red rectangle onto the backend: 20 21 ```rust 22 use std::iter::{Once, once}; 23 use plotters::element::{PointCollection, Drawable}; 24 use plotters_backend::{BackendCoord, DrawingErrorKind, BackendStyle}; 25 use plotters::style::IntoTextStyle; 26 use plotters::prelude::*; 27 28 // Any example drawing a red X 29 struct RedBoxedX((i32, i32)); 30 31 // For any reference to RedX, we can convert it into an iterator of points 32 impl <'a> PointCollection<'a, (i32, i32)> for &'a RedBoxedX { 33 type Point = &'a (i32, i32); 34 type IntoIter = Once<&'a (i32, i32)>; 35 fn point_iter(self) -> Self::IntoIter { 36 once(&self.0) 37 } 38 } 39 40 // How to actually draw this element 41 impl <DB:DrawingBackend> Drawable<DB> for RedBoxedX { 42 fn draw<I:Iterator<Item = BackendCoord>>( 43 &self, 44 mut pos: I, 45 backend: &mut DB, 46 _: (u32, u32), 47 ) -> Result<(), DrawingErrorKind<DB::ErrorType>> { 48 let pos = pos.next().unwrap(); 49 backend.draw_rect(pos, (pos.0 + 10, pos.1 + 12), &RED, false)?; 50 let text_style = &("sans-serif", 20).into_text_style(&backend.get_size()).color(&RED); 51 backend.draw_text("X", text_style, pos) 52 } 53 } 54 55 fn main() -> Result<(), Box<dyn std::error::Error>> { 56 let root = BitMapBackend::new( 57 "plotters-doc-data/element-0.png", 58 (640, 480) 59 ).into_drawing_area(); 60 root.draw(&RedBoxedX((200, 200)))?; 61 Ok(()) 62 } 63 ``` 64 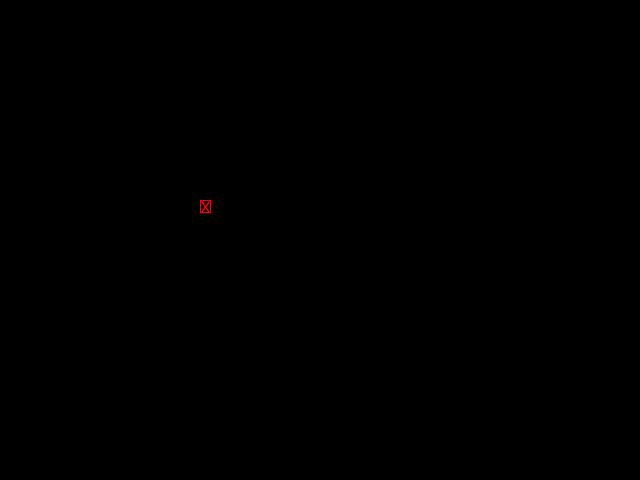 65 66 ## Composable Elements 67 You also have an convenient way to build an element that isn't built into the Plotters library by 68 combining existing elements into a logic group. To build an composable element, you need to use an 69 logic empty element that draws nothing to the backend but denotes the relative zero point of the logical 70 group. Any element defined with pixel based offset coordinate can be added into the group later using 71 the `+` operator. 72 73 For example, the red boxed X element can be implemented with Composable element in the following way: 74 ```rust 75 use plotters::prelude::*; 76 fn main() -> Result<(), Box<dyn std::error::Error>> { 77 let root = BitMapBackend::new( 78 "plotters-doc-data/element-1.png", 79 (640, 480) 80 ).into_drawing_area(); 81 let font:FontDesc = ("sans-serif", 20).into(); 82 root.draw(&(EmptyElement::at((200, 200)) 83 + Text::new("X", (0, 0), &"sans-serif".into_font().resize(20.0).color(&RED)) 84 + Rectangle::new([(0,0), (10, 12)], &RED) 85 ))?; 86 Ok(()) 87 } 88 ``` 89 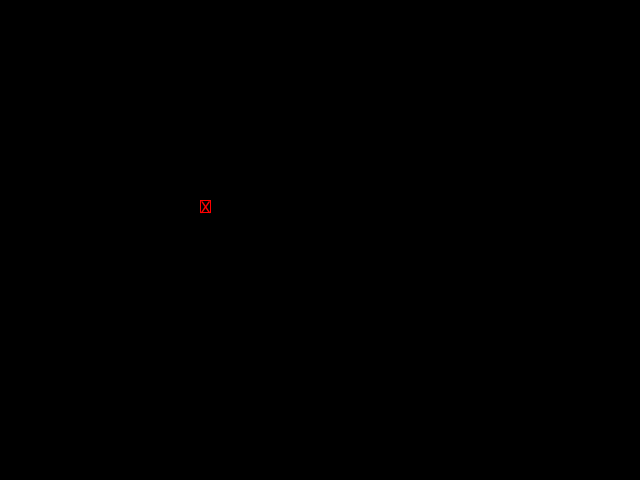 90 91 ## Dynamic Elements 92 By default, Plotters uses static dispatch for all the elements and series. For example, 93 the `ChartContext::draw_series` method accepts an iterator of `T` where type `T` implements 94 all the traits a element should implement. Although, we can use the series of composable element 95 for complex series drawing. But sometimes, we still want to make the series heterogynous, which means 96 the iterator should be able to holds elements in different type. 97 For example, a point series with cross and circle. This requires the dynamically dispatched elements. 98 In plotters, all the elements can be converted into `DynElement`, the dynamic dispatch container for 99 all elements (include external implemented ones). 100 Plotters automatically implements `IntoDynElement` for all elements, by doing so, any dynamic element should have 101 `into_dyn` function which would wrap the element into a dynamic element wrapper. 102 103 For example, the following code counts the number of factors of integer and mark all prime numbers in cross. 104 ```rust 105 use plotters::prelude::*; 106 fn num_of_factor(n: i32) -> i32 { 107 let mut ret = 2; 108 for i in 2..n { 109 if i * i > n { 110 break; 111 } 112 113 if n % i == 0 { 114 if i * i != n { 115 ret += 2; 116 } else { 117 ret += 1; 118 } 119 } 120 } 121 return ret; 122 } 123 fn main() -> Result<(), Box<dyn std::error::Error>> { 124 let root = 125 BitMapBackend::new("plotters-doc-data/element-3.png", (640, 480)) 126 .into_drawing_area(); 127 root.fill(&WHITE)?; 128 let mut chart = ChartBuilder::on(&root) 129 .x_label_area_size(40) 130 .y_label_area_size(40) 131 .margin(5) 132 .build_ranged(0..50, 0..10)?; 133 134 chart 135 .configure_mesh() 136 .disable_x_mesh() 137 .disable_y_mesh() 138 .draw()?; 139 140 chart.draw_series((0..50).map(|x| { 141 let center = (x, num_of_factor(x)); 142 // Although the arms of if statement has different types, 143 // but they can be placed into a dynamic element wrapper, 144 // by doing so, the type is unified. 145 if center.1 == 2 { 146 Cross::new(center, 4, Into::<ShapeStyle>::into(&RED).filled()).into_dyn() 147 } else { 148 Circle::new(center, 4, Into::<ShapeStyle>::into(&GREEN).filled()).into_dyn() 149 } 150 }))?; 151 152 Ok(()) 153 } 154 ``` 155 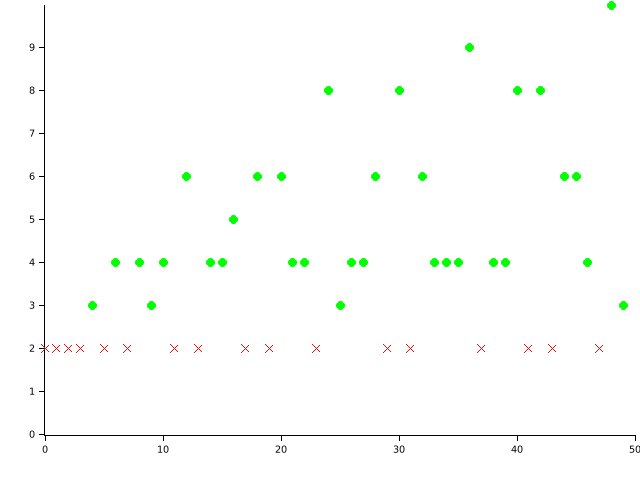 156 */ 157 use plotters_backend::{BackendCoord, DrawingBackend, DrawingErrorKind}; 158 use std::borrow::Borrow; 159 160 mod basic_shapes; 161 pub use basic_shapes::*; 162 163 mod text; 164 pub use text::*; 165 166 mod points; 167 pub use points::*; 168 169 mod composable; 170 pub use composable::{ComposedElement, EmptyElement}; 171 172 #[cfg(feature = "candlestick")] 173 mod candlestick; 174 #[cfg(feature = "candlestick")] 175 pub use candlestick::CandleStick; 176 177 #[cfg(feature = "errorbar")] 178 mod errorbar; 179 #[cfg(feature = "errorbar")] 180 pub use errorbar::{ErrorBar, ErrorBarOrientH, ErrorBarOrientV}; 181 182 #[cfg(feature = "boxplot")] 183 mod boxplot; 184 #[cfg(feature = "boxplot")] 185 pub use boxplot::Boxplot; 186 187 #[cfg(feature = "bitmap_backend")] 188 mod image; 189 #[cfg(feature = "bitmap_backend")] 190 pub use self::image::BitMapElement; 191 192 mod dynelem; 193 pub use dynelem::{DynElement, IntoDynElement}; 194 195 /// A type which is logically a collection of points, under any given coordinate system. 196 /// Note: Ideally, a point collection trait should be any type of which coordinate elements can be 197 /// iterated. This is similar to `iter` method of many collection types in std. 198 /// 199 /// ```ignore 200 /// trait PointCollection<Coord> { 201 /// type PointIter<'a> : Iterator<Item = &'a Coord>; 202 /// fn iter(&self) -> PointIter<'a>; 203 /// } 204 /// ``` 205 /// 206 /// However, 207 /// [Generic Associated Types](https://github.com/rust-lang/rfcs/blob/master/text/1598-generic_associated_types.md) 208 /// is far away from stablize. 209 /// So currently we have the following workaround: 210 /// 211 /// Instead of implement the PointCollection trait on the element type itself, it implements on the 212 /// reference to the element. By doing so, we now have a well-defined lifetime for the iterator. 213 /// 214 /// In addition, for some element, the coordinate is computed on the fly, thus we can't hard-code 215 /// the iterator's return type is `&'a Coord`. 216 /// `Borrow` trait seems to strict in this case, since we don't need the order and hash 217 /// preservation properties at this point. However, `AsRef` doesn't work with `Coord` 218 /// 219 /// This workaround also leads overly strict lifetime bound on `ChartContext::draw_series`. 220 /// 221 /// TODO: Once GAT is ready on stable Rust, we should simplify the design. 222 /// 223 pub trait PointCollection<'a, Coord> { 224 /// The item in point iterator 225 type Point: Borrow<Coord> + 'a; 226 227 /// The point iterator 228 type IntoIter: IntoIterator<Item = Self::Point>; 229 230 /// framework to do the coordinate mapping point_iter(self) -> Self::IntoIter231 fn point_iter(self) -> Self::IntoIter; 232 } 233 /// The trait indicates we are able to draw it on a drawing area 234 pub trait Drawable<DB: DrawingBackend> { 235 /// Actually draws the element. The key points is already translated into the 236 /// image coordinate and can be used by DC directly draw<I: Iterator<Item = BackendCoord>>( &self, pos: I, backend: &mut DB, parent_dim: (u32, u32), ) -> Result<(), DrawingErrorKind<DB::ErrorType>>237 fn draw<I: Iterator<Item = BackendCoord>>( 238 &self, 239 pos: I, 240 backend: &mut DB, 241 parent_dim: (u32, u32), 242 ) -> Result<(), DrawingErrorKind<DB::ErrorType>>; 243 } 244